3D Objects
Working with 3D content
TODO:
- Broken developer.viromedia.com links
- Is "Support for other platforms" on the way?
- incoming glTF features?
The Object3D component enables loading 3D objects (also known as models or meshes) into a scene.
Object3D defines the structure of the 3D object: its vertices, faces, and texture coordinates. The scene graph, or Node hierarchy, determines the orientation and placement of the object. And finally, the appearance of the Object3D is defined by its materials.
Loading 3D Objects
Viro supports loading 3D models in FBX, OBJ, and GLTF formats. Viro will load the geometry, textures, and lighting parameters in the file. Installed skeletal animations will also be loaded automatically for FBX models, with this support coming soon for GLTF (which currently supports static models only). OBJ and GLTF files can be fed directly into Object3D, while FBX files need to converted into Viro's own VRX format.
3D models may be loaded from any URI. To load assets in the application bundle, use URIs of the form file:///android_asset/[asset-name]
. Models are always loaded asynchronously into the Object3D. The following snippet shows how a model of the human heart would be loaded.
Object3D heart = new Object3D();
heart.loadModel(Uri.parse("file:///android_asset/heart.obj"), Object3D.Type.OBJ, new AsyncObject3DListener() {
public void onObject3DFailed(String error) {
Log.w(TAG, "Failed to load the model");
}
public void onObject3DLoaded(Object3D object, Object3D.Type type)
Log.i(TAG, "Successfully loaded the model!");
}
});
Scene scene = new Scene();
scene.getRootNode().addChildNode(heart);
Orientation and Placement
The node hierarchy and its transforms determine how 3D objects are positioned in the scene. See the Scene Graph guide for more information.
Materials
Materials define the appearance of 3D objects. Materials control how an object responds to light. For detailed information see the Lighting and Materials guide.
For OBJ models, materials can either be explicitly set via Object3D.getGeometry().setMaterials(List<Material>)
, or they can be loaded via an MTL file. MTL is an extension of the OBJ file format, that allows material properties to be set for each surface of a model. To load an OBJ with an associated MTL file, the MTL file and all of its associated textures must placed in the same directory as the OBJ file. They will then be automatically retrieved and applied.
Callbacks
Model loading is performed asynchronously, so as not to introduce lag into your application. Viro provides callbacks through [AsyncObject3DListener](https://developer.viromedia.com/virocore/reference/com/viro/core/AsyncObject3DListener.html] to respond to the model loading process.
FBX
FBX is an expansive and flexible 3D model format supported by most 3D authoring software. To load FBX files, use the ViroFBX script to convert the FBX file into a VRX file. The VRX file can then be loaded using Object3D.
MacOS X Support Only
Currently the ViroFBX tool runs only on Mac OS X. Support for other platforms is on the way.
The ViroFBX script can be found here.
The following example shows how to convert a model into VRX format:
./ViroFBX path/to/model.fbx path/to/model.vrx
In the example above, path/to/model.fbx
is the path to the FBX file to convert, and path/to/model.vrx
is the path to the VRX file to create. Once the VRX file is created, it can be loaded into an application with Object3D. Again, remember to place the model's associated textures in the same directory as the VRX file. Below is a simple example for loading a VRX file.
Object3D model = new Object3D();
model.loadModel(Uri.parse("file:///android_asset/model.vrx"), Object3D.Type.FBX, new AsyncObject3DListener() {
public void onObject3DFailed(String error) {
Log.w(TAG, "Failed to load the model");
}
public void onObject3DLoaded(Object3D object, Object3D.Type type)
Log.i(TAG, "Successfully loaded the model!");
}
});
Scene scene = new Scene();
scene.getRootNode().addChildNode(model);
Model Not Appearing?
You may need to add a Light to your scene. Try adding an Ambient light to see if your model appears:
AmbientLight ambient = new AmbientLight(Color.WHITE, 1000.0f); node.addLight(ambient);
See the Lighting and Materials) guide for more details.
Skeletal Animation
FBX models support skeletal and keyframe animation. Skeletal animation is a hierarchical technique for animating complex geometries like humanoids. Keyframe animation is a technique for chaining together smooth transitions by their start and end points. Viro automatically loads all skeletal and keyframe animations into the Object3D.
To run these animations, retrieve the animation via Node.getAnimation(String)
. Using the Animation object, you can then configure the animation and run it. For example, if the FBX file had an animation called "Take 001", you could run it as follows:
Object3D model = new Object3D();
model.loadModel(Uri.parse("file:///android_asset/model.vrx"), Object3D.Type.FBX, new AsyncObject3DListener() {
public void onObject3DFailed(String error) {
Log.w(TAG, "Failed to load the model");
}
public void onObject3DLoaded(Object3D object, Object3D.Type type)
// Now that the model is loaded, get its animation
Animation animation = object.getAnimation("Take 001");
// Make the animation loop, then play it
animation.setLoop(true);
animation.play();
}
});
Scene scene = new Scene();
scene.getRootNode().addChildNode(model);
For more information on animations, see the Animation guide.
GLTF
The GL Transmission Format (glTF) is an API-neutral runtime asset delivery format. glTF bridges the gap between 3D content creation tools and modern 3D applications by providing an efficient, extensible, and interoperable format for the transmission and loading of 3D content. Viro currently supports gLTF 2.0.
glTF Model Format
glTF assets are represented by the following 3 subcomponents:
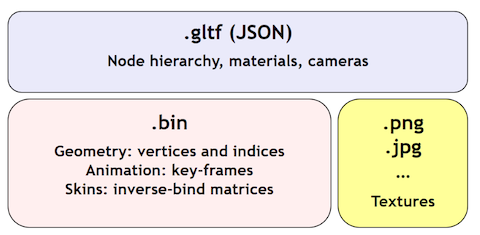
- A JSON-formatted file (.gltf) containing a full scene description: node hierarchy, materials, cameras, as well as descriptor information for meshes, animations, and other constructs
- Binary files (.bin) containing geometry and animation data, and other buffer-based data
- Image files (.jpg, .png) for textures
Loading glTF Models
There are 3 ways you can provide your glTF data to Viro to be rendered:
- Import the basic 3 components individually: The .gltf, its corresponding binary, and its image files.
- Import a single .gltf file, where both the binary and image data are embedded into the .gltf file as Base64-encoded data.
- Import a single .glb file that represents all required glTF components in a raw binary format.
Like FBX, you can use ViroCore's Object3D component and the loadModel() API to load a glTF model into your scene. When loading glTF files, set the Object3D.Type to either Object3D.Type.GLTF
(for options 1 and 2 above), or Object3D.Type.GLB
(for option 3: raw glTF binary).
It is also important to note that any related glTF assets (like binary or image files) are always referenced by the URIs specified within the glTF file. Relative paths are with respect to the location of the glTF file.
Incoming glTF features and known limitations
At the moment, Viro supports rendering static 2.0 glTF models. We are also currently in the process of implementing the full support for the complete set of gLTF features, so stay tuned! :)
Incoming features includes:
- Support for additional gLTF Extensions.
- Emissive Maps
- Sparse Accessor Data Formats
- Non-indexed mesh rendering (drawArray rendering)
- Additional Primitive Modes (Line_Loop & Triangle_Fan)
- Material Alpha Mask Mode
- Additional Min / Mag Filter modes
- Double sided rendering
- Baked in gLTF Camera
Updated about 3 years ago